Finite State Machines in Game Development
Finite State Machines (FSM) are a powerful tool for managing complex behaviors in game development. They allow developers to create organized and predictable systems, making it easier to handle various game states and transitions. This article will cover the basics of FSMs, their applications in game development, and a step-by-step guide to implementing an FSM in Unity.
What is a Finite State Machine?
A Finite State Machine is a computational model used to design algorithms. It consists of a finite number of states, transitions between those states, and actions that occur due to those transitions. FSMs are used to manage the states of objects, characters, or systems within a game, ensuring that each entity behaves predictably.
Key Components of an FSM
1. States: Different conditions or modes of the system (e.g., Idle, Walking, Attacking).
2. Transitions: Conditions that trigger a change from one state to another (e.g., player input, timer).
3. Actions: Activities performed during a state or transition (e.g., animations, movements).
Applications in Game Development
FSMs are widely used in game development for:
Character Behavior: Managing AI states like patrolling, chasing, and attacking.
Game States: Handling different phases of a game such as menus, gameplay, and game over screens.
Animations: Controlling animation sequences based on character states.
Event Systems: Triggering events based on game states.
Implementing an FSM in Unity
Let’s walk through the implementation of an FSM in Unity using C#. We will create a simple FSM to manage a character’s states: Idle, Walking, and Attacking.
Step 1: Define the State Base Class
First, create an abstract base class for the states:
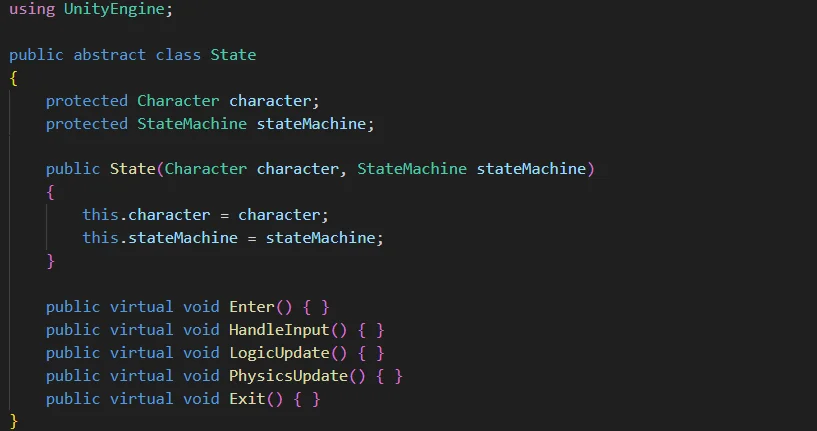
Step 2: Implement Specific States
Next, create specific states inheriting from the base class:
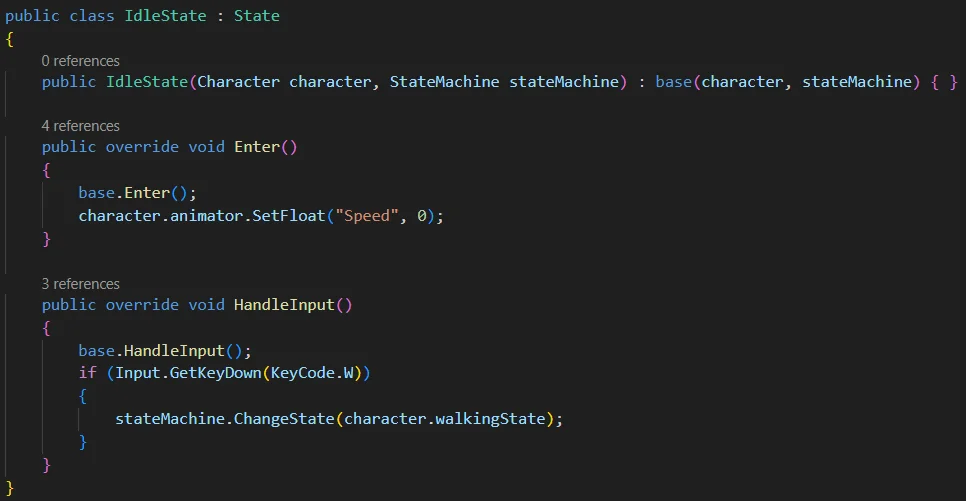
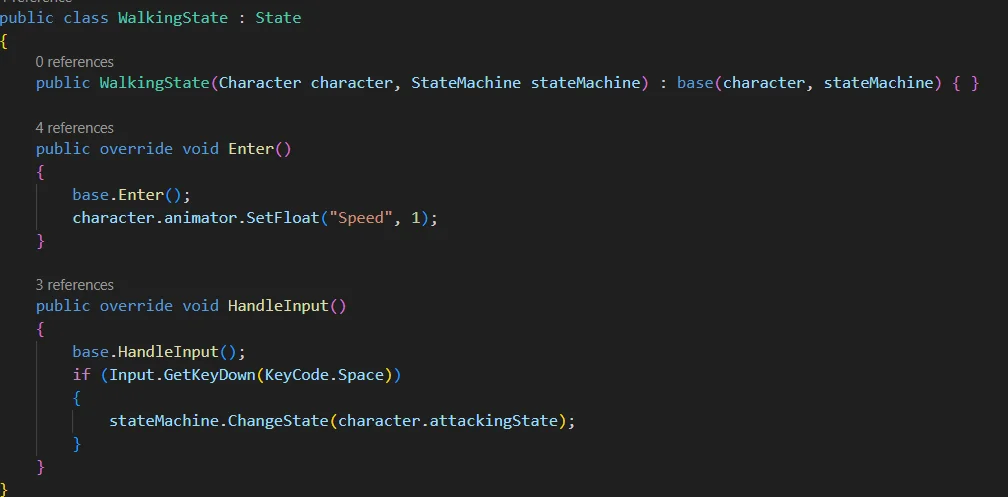
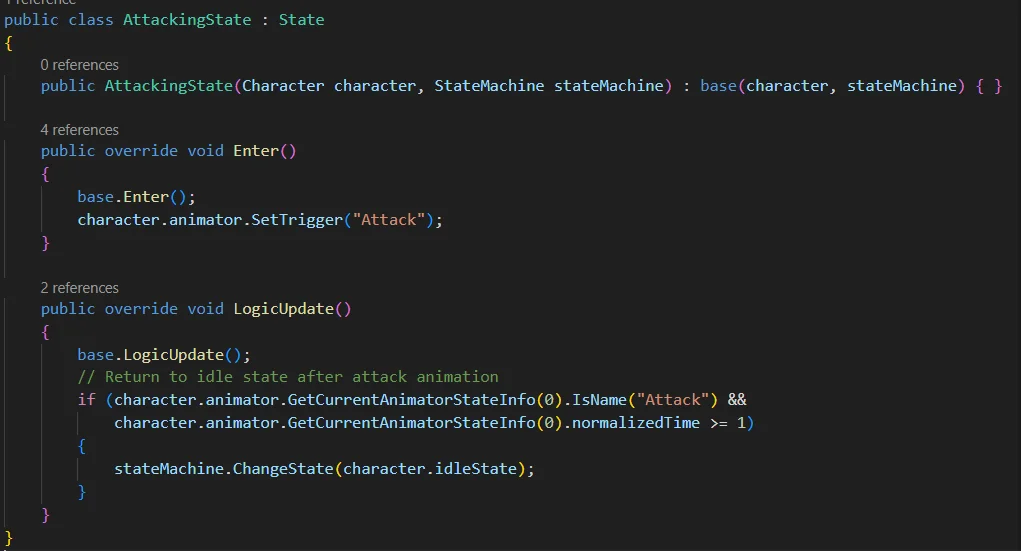
Step 3: Create the State Machine
Create the StateMachine class to manage transitions:
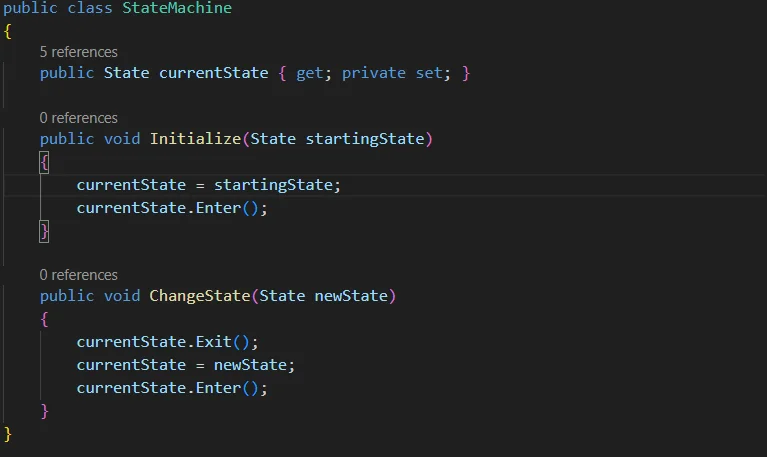
Step 4: Integrate with Character
Modify the Character class to integrate with the FSM:
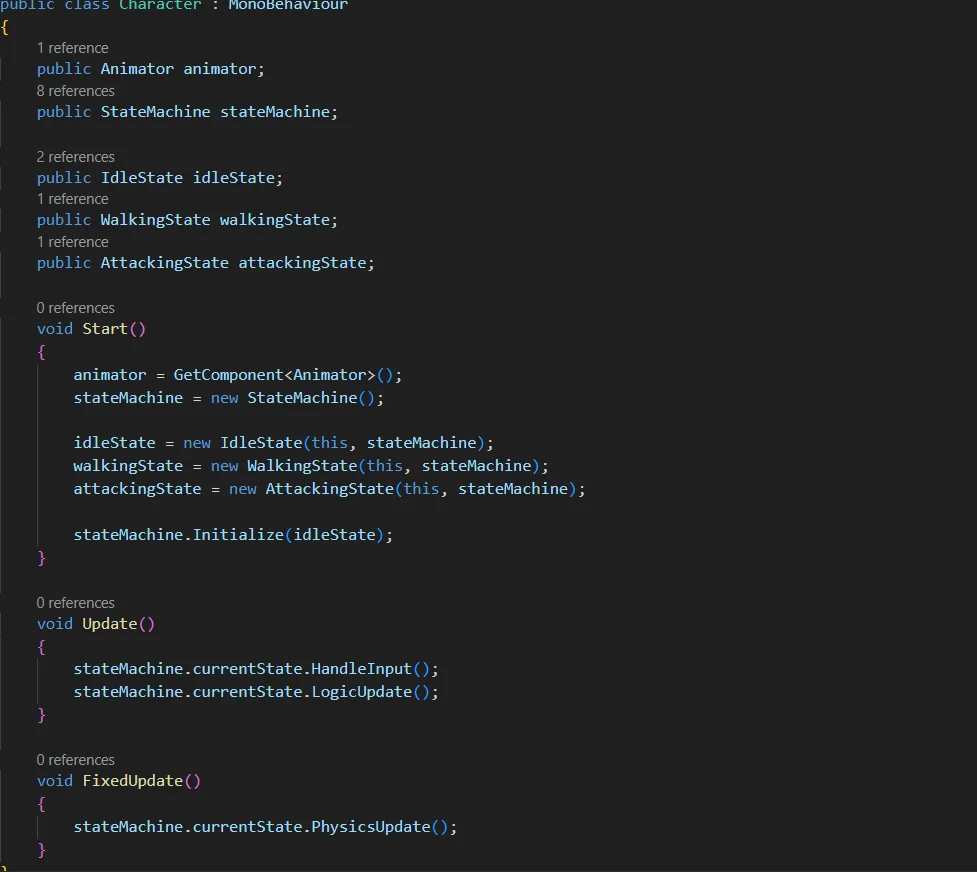
Conclusion
Finite State Machines are essential for managing complex behaviors in games. They help in organizing code, making systems more predictable, and simplifying debugging. By following the steps outlined above, you can implement FSMs in Unity to control character behaviors, game states, and more. This structured approach will enhance your game’s maintainability and scalability, providing a better experience for players and developers alike.
This is just a simple example of using FSM in your game. You may change the process according to your preferences!
Cheers!